Today I discuss some functionality about Angularjs. This is the most popular javascript framework. In this article. I give some code snippet with Angularjs. This code helps to you write to UI Data Add, Edit, Delete with real-time updates.
First, we need Angularjs link
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
After then we will be writing some HTML codes.
<table ng-controller="ctrl" style="width:100%"> <tr> <th>Name</th> <th>Address</th> <th>Actions</th> </tr> <tr ng-repeat="user in users"> <td>{{ user.name }}</td> <td>{{ user.addr }}</td> <td><button ng-click="edit(user)">Edit</button> | <button ng-click="delete('{{user.name}}')">Delete</button></td> </tr> </table> <input type="text" ng-model="name" placeholder="Input Name" /> <input type="text" ng-model="addr" placeholder="Input Address" /> <button ng-click="add()">Add</button> <button ng-click="save()">Save</button>
After this code, we will be writing js codes to implements CRUD functionality.
var app = angular.module('app',[]); app.controller('ctrl',function($scope,$rootScope, $log){ $scope.users = [ {name:'Dipyan',addr:'Birati'}, {name:'Shibaji',addr:'Bishorpara'} ]; $rootScope.add = function(){ if(angular.isDefined($rootScope.name) && angular.isDefined($rootScope.addr) && $rootScope.name!='' && $rootScope.addr !='') $scope.users.push({name: $rootScope.name, addr: $rootScope.addr}); $rootScope.name =''; $rootScope.addr =''; $log.log('Add Function Works'); } $scope.delete = function(val){ $scope.users.splice(val,1); } $scope.edit = function(val){ $rootScope.id = val.name; $rootScope.name = val.name; $rootScope.addr = val.addr; } $rootScope.save = function(){ for(var i in $scope.users){ if($scope.users[i].name == $rootScope.id){ $scope.users[i].name = $rootScope.name; $scope.users[i].addr = $rootScope.addr; } } $rootScope.name =''; $rootScope.addr =''; } });
After this written, we will get output like this.
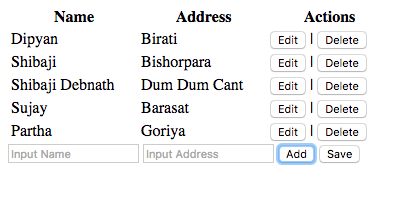
Now, we are the discussion about codes. Which codes usages here and why I am written that codes?
First, when we are writing Angularjs codes that time we need to initiate the “APP” directives.
in HTML
<div ng-app="APP"> .... </div>
This is the main root directive. after then we need to bind this directive with JS code.
var app = angular.module('APP',[]);
After this, we need to be setup “Ctrl” directive for visualising the area scope. So codes will be
in Html
<div ng-app='APP'> <div ng-controller='Ctrl'> .... </div> </div>
in JavaScript
var app = angular.module('APP', []); app.controller('Ctrl', function(){ ... });
Now we will create a table to represent the data. So we will be written this codes.
<table ng-controller="ctrl" style="width:100%"> <tr> <th>Name</th> <th>Address</th> <th>Actions</th> </tr> <tr ng-repeat="user in users"> <td>{{ user.name }}</td> <td>{{ user.addr }}</td> <td><button ng-click="edit(user)">Edit</button> | <button ng-click="delete('{{user.name}}')">Delete</button></td> </tr> </table>
Here I am using ng-controller to visualise the data from the model. this is coming from the server database or REST API. Basically, we get data access from the server through js model which is controlling by the angular module. But here I am given only static procedures. Because server site will be different technology for generating the REST API. If you want to access the REST API for it. You can follow my previous article AJAX Calling by AngularJS Here I made a real-time currency exchange api integrate with the apps.
ng-repeat=”user in users” this directive can be accessing the data with “users” array objects from there.
I hope, it will help who are wanted to some interest on AngularJS.